Image Preprocessing#
Dataset source: https://sipi.usc.edu/database/database.php?volume=misc
!poetry add opencv-python
The following packages are already present in the pyproject.toml and will be skipped:
- opencv-python
If you want to update it to the latest compatible version, you can use `poetry update package`.
If you prefer to upgrade it to the latest available version, you can use `poetry add package@latest`.
Nothing to add.
from matplotlib import pyplot as plt
def display_cv2_image(img):
plt.imshow(img, cmap="gray")
plt.show()
Loading and converting images#
import cv2
img = cv2.imread("../../data/peppers.tiff")
display_cv2_image(img)
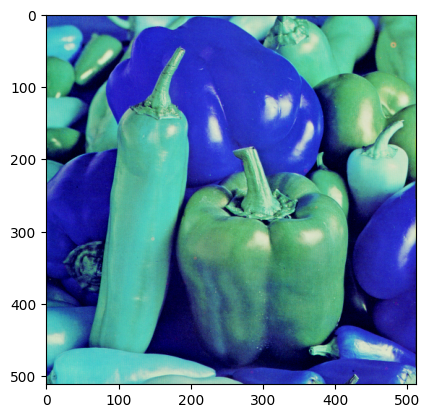
Converting between color spaces#
rgb_img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
display_cv2_image(rgb_img)
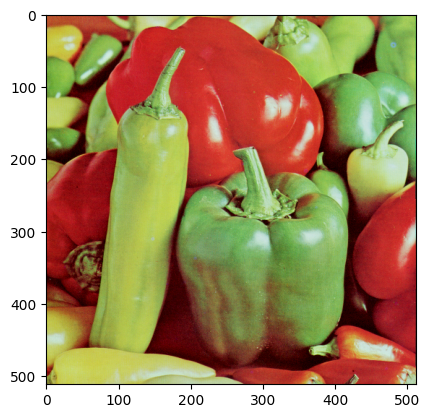
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
display_cv2_image(gray_img)
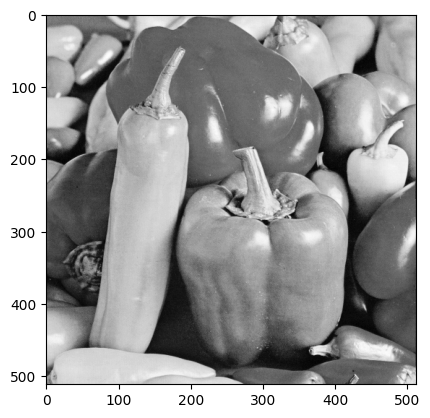
Resizing and cropping images#
resized = cv2.resize(rgb_img, (100, 100))
display_cv2_image(resized)
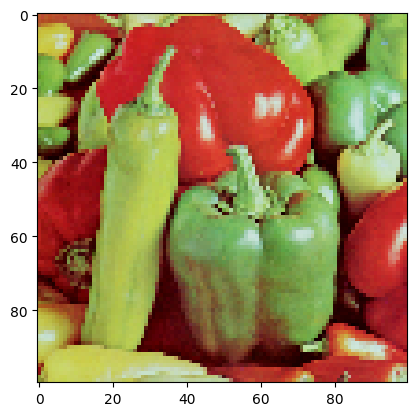
x1, y1 = 100, 100 # Top-left corner
x2, y2 = 400, 400 # Bottom-right corner
cropped = rgb_img[y1:y2, x1:x2]
display_cv2_image(cropped)
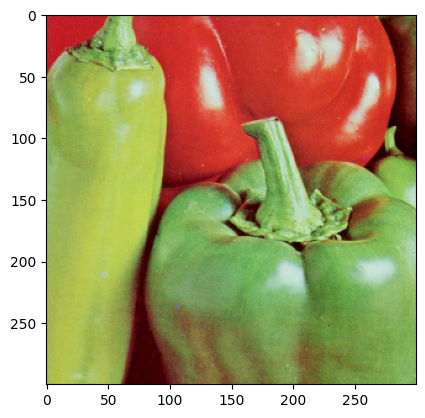